Android App Developer Program: A Comprehensive Guide to Building Mobile Apps
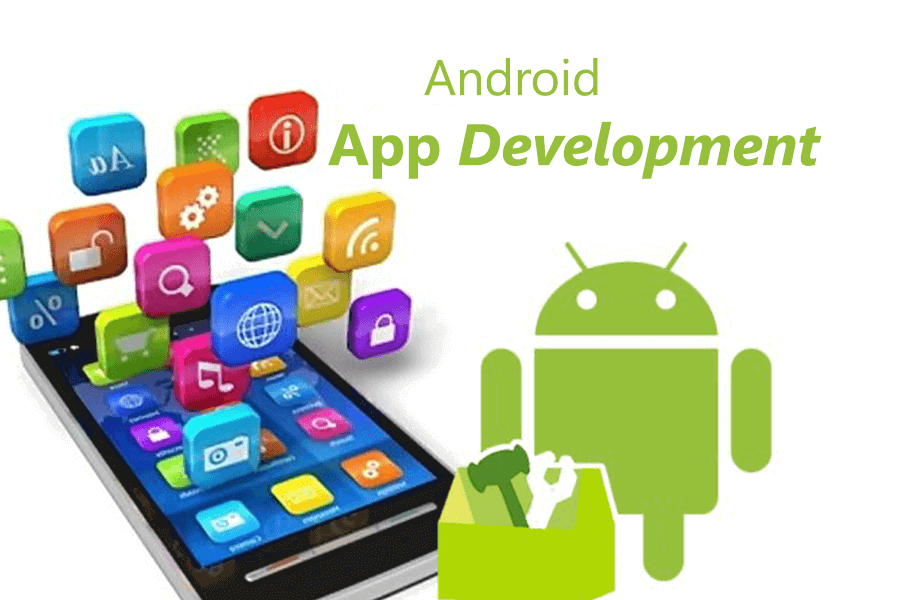
Are you an aspiring Android app developer looking to break into the world of mobile app development? Look no further! In this blog article, we will provide you with a comprehensive guide to the Android App Developer Program, covering everything from the basics to advanced techniques. Whether you are a beginner or an experienced developer, this article will equip you with the knowledge and resources you need to create high-quality Android apps.
Before we dive into the nitty-gritty details, let's start with an overview of the Android App Developer Program. Developed by Google, this program offers a range of tools, resources, and support to help developers create, test, and distribute their applications on the Android platform. With a vast user base and a thriving app ecosystem, Android provides an incredible opportunity for developers to reach millions of users worldwide.
Understanding the Android App Development Environment
In this section, we will explore the Android app development environment, including the software development kit (SDK), Integrated Development Environment (IDE), and essential tools. We will also discuss setting up your development environment and getting started with your first Android app.
1. Software Development Kit (SDK)
The Android SDK is a collection of software tools and libraries that developers use to create Android apps. It includes a comprehensive set of APIs (Application Programming Interfaces) that allow developers to access various features and functionalities of the Android platform. The SDK also provides emulators and debugging tools to test and debug apps.
To get started, you will need to download and install the Android SDK on your computer. Once installed, you can use the SDK Manager to download specific versions of Android platforms, tools, and other components required for app development.
2. Integrated Development Environment (IDE)
An Integrated Development Environment (IDE) is a software application that provides a complete development environment for writing, testing, and debugging code. Android Studio is the official IDE for Android app development, developed by Google. It offers a rich set of features, such as code editing, debugging, and layout design tools, specifically tailored for Android development.
When starting a new project, Android Studio provides project templates, which include the necessary files and folder structure to kickstart your app development. It also integrates with the Android SDK, allowing seamless access to various resources and tools.
3. Essential Tools
Alongside the SDK and IDE, several essential tools can enhance your Android app development experience. These tools help streamline the development process, improve efficiency, and aid in debugging and testing. Some of the key tools include:
- Android Debug Bridge (ADB): A versatile command-line tool that allows you to communicate with a connected Android device or emulator. With ADB, you can install and uninstall apps, access device logs, and execute various commands.
- Android Virtual Device (AVD) Manager: This tool enables you to create and manage virtual devices for testing your app on different Android versions and device configurations. It provides a simulated environment to ensure your app works seamlessly across various devices.
- Android Asset Packaging Tool (AAPT): AAPT allows you to compile and package resources, such as images, XML files, and other assets, into the APK (Android Package) file format. It performs resource validation and optimization, ensuring your app is efficient and optimized.
- ProGuard: ProGuard is a tool that helps optimize and obfuscate your app's code, making it harder for reverse engineering and unauthorized access. It removes unused code, shrinks the code size, and obfuscates class and method names.
4. Setting Up Your Development Environment
Before you start coding your Android app, you need to set up your development environment. Here are the steps to follow:
- Download and install the latest version of Android Studio from the official website.
- Launch Android Studio and follow the on-screen instructions to complete the installation process.
- Once installed, open Android Studio and set the SDK path by going to File > Project Structure > SDK Location. Here, you can specify the path to your Android SDK installation.
- Create a new project by selecting File > New > New Project. Choose the project template, set the project name, package name, and other relevant details.
- Select the minimum SDK version and target SDK version based on your app's requirements.
- Click "Finish" to create your project.
Congratulations! You have successfully set up your development environment and are ready to start building your Android app.
Java Fundamentals for Android Development
Java is the primary programming language used for Android app development. In this section, we will cover the essential Java concepts and techniques that every Android developer should know. From variables and control flow to object-oriented programming, this session will lay the foundation for your Android development journey.
1. Variables and Data Types
Variables are used to store data in memory during runtime. In Java, you need to declare variables before using them. Each variable has a specific data type, such as int, float, or String, which determines the kind of data it can hold. Understanding the different data types and how to declare and initialize variables is crucial for writing efficient and bug-free code.
For example, to declare an integer variable named "age" and assign it a value of 25, you would write:
int age = 25;
Similarly, you can declare variables of other data types, such as float, double, boolean, and String.
2. Operators and Expressions
Operators are symbols that perform various operations on variables and values. They can be used for arithmetic calculations, logical comparisons, and more. Understanding operators and how to use them in expressions is essential for manipulating data and making decisions in your app.
For example, the "+" operator is used for addition, the "-" operator for subtraction, and the "==" operator for equality comparison. You can combine multiple operators and operands to form complex expressions.
3. Control Flow Statements
Control flow statements allow you to control the execution flow of your program based on certain conditions. They include if-else statements, switch statements, and looping constructs like for loops and while loops. These statements help you make decisions, repeat tasks, and handle different scenarios in your app.
For example, an if-else statement allows you to execute a block of code if a certain condition is true, and a different block of code if the condition is false. This helps you create dynamic and responsive apps based on user input and other factors.
4. Object-Oriented Programming (OOP)
Object-Oriented Programming (OOP) is a programming paradigm that revolves around the concept of objects. In Java, everything is an object, and objects are instances of classes. OOP allows you to organize your code into reusable and modular units, making it easier to manage and maintain.
Key OOP concepts in Java include classes, objects, inheritance, polymorphism, and encapsulation. Classes serve as blueprints for objects, defining their properties (attributes) and behaviors (methods). Inheritance allows you to create new classes based on existing ones, inheriting their properties and behaviors. Polymorphism enables objects to take on multiple forms, depending on the context. Encapsulation encapsulates data and methods within a class, ensuring data security and code organization.
5. Exception Handling
Exception handling is the process of handling runtime errors and exceptional conditions in your app. Java provides a robust exception handling mechanism that allows you to catch and handle exceptions gracefully, preventing app crashes and improving user experience.
With try-catch blocks, you can catch and handle specific exceptions, such as divide-by-zero errors or file-not-found errors. You can also create custom exceptions to handle application-specific errors. Proper exception handling ensures your app can recover from errors and provides meaningful error messages to users.
6. File I/O and Serialization
Many Android apps require reading from and writing to files, such as storing user preferences or saving data locally. Java provides classes and APIs for file input/output (I/O) operations, allowing you to read and write data to files and directories on the device's storage.
Serialization is the process of converting objects into a byte stream, which can be saved to a file or transmitted over a network. Java provides serialization mechanisms that allow you to save and restore object states, enabling data persistence and transfer.
User Interface Design and Layouts
A well-designed user interface is crucial for the success of any Android app. In this section, we will explore the various UI components and layout options available in Android. We will also delve into responsive design principles and best practices to create visually appealing and user-friendly interfaces.
1. UI Components and Widgets
Android provides a rich set of UI components and widgets that you can use to build your app's user interface. These components include buttons, text fields, checkboxes, radio buttons, spinners, and more. Understanding how to use these components and customize their appearance and behavior is essentialto creating a visually appealing and interactive user interface.
Each UI component has its own set of properties and methods that allow you to modify its appearance and handle user interactions. For example, you can set the text and background color of a button, define the options for a spinner, or handle a button click event. By leveraging these components effectively, you can create intuitive and user-friendly interfaces that enhance the overall user experience.
2. XML Layouts
In Android, user interfaces are typically defined using XML (Extensible Markup Language) layouts. XML layouts provide a declarative way to describe the structure and appearance of your app's UI elements. This separation of UI and logic allows for easier maintenance and collaboration between designers and developers.
Android provides several layout types, including LinearLayout, RelativeLayout, ConstraintLayout, and FrameLayout. Each layout type offers different ways to position and arrange UI components on the screen. For example, LinearLayout arranges components linearly in either a vertical or horizontal orientation, while RelativeLayout allows you to position components relative to each other or the parent layout.
By nesting layouts and using layout attributes, you can create complex UI designs and achieve the desired visual hierarchy. XML layouts also support attributes for specifying dimensions, margins, padding, and more, giving you fine-grained control over the appearance and behavior of your UI.
3. Responsive Design and Screen Sizes
With the wide variety of Android devices available, it's essential to design your app to be responsive and adaptable to different screen sizes and resolutions. Responsive design ensures that your app's UI elements are appropriately sized and positioned, regardless of the device's screen size or orientation.
Android provides several techniques for achieving responsive design. One approach is to use density-independent pixels (dp) instead of regular pixels (px) for defining dimensions in your layouts. By using dp, your UI elements will scale proportionally based on the device's screen density, ensuring a consistent user experience across different devices.
In addition to using dp, you can also use layout constraints to create responsive layouts. ConstraintLayout, introduced in Android Studio 2.2, allows you to define flexible constraints between UI components, enabling them to adapt and resize based on the available space. This constraint-based layout system provides powerful tools for creating responsive and dynamic UI designs.
4. Material Design Guidelines
Google's Material Design guidelines provide a comprehensive set of design principles and best practices for creating visually appealing and intuitive user interfaces. Material Design emphasizes a clean and minimalistic aesthetic, with a focus on bold colors, typography, and meaningful animations.
By following the Material Design guidelines, you can ensure that your app's UI aligns with the overall Android design language, creating a cohesive and familiar experience for users. The guidelines cover various aspects of UI design, including layout structure, color palettes, typography, icons, and motion design.
Some key principles of Material Design include:
- Elevation and Depth: Material Design introduces a concept of elevation, which gives a sense of depth and hierarchy to UI elements. By applying elevation values to different components, you can create a visually appealing and interactive user interface.
- Responsive Animations: Material Design encourages the use of meaningful animations to provide feedback and enhance the user experience. Animations can be used to convey transitions between screens, highlight interactions, or provide visual cues.
- Color and Typography: Material Design provides a predefined color palette and typography guidelines, ensuring consistency and readability across different devices and screen sizes.
- Iconography: Material Design offers a set of standardized icons that can be used to represent various actions and elements in your app. These icons follow a consistent visual language and are scalable to different sizes.
By incorporating Material Design principles into your app's UI, you can create a visually appealing and cohesive user experience that aligns with the modern Android design language.
Working with Data and Databases
Most apps require the storage and management of data. In this section, we will discuss the different data storage options available, including shared preferences, files, and databases. We will also cover SQLite, a lightweight and efficient database engine commonly used in Android app development.
1. Shared Preferences
Shared preferences provide a simple and lightweight way to store key-value pairs of data. It is ideal for storing small amounts of data, such as user preferences, settings, or app preferences. Shared preferences are stored as XML files in the app's private storage area and can be accessed throughout the app.
To work with shared preferences, you need to create an instance of the SharedPreferences class and use its methods to read, write, and delete key-value pairs. SharedPreferences allows you to store data of various types, such as strings, booleans, integers, and floats.
For example, to store a user's name in shared preferences:
// Get the shared preferences instanceSharedPreferences sharedPreferences = getSharedPreferences("MyPrefs", Context.MODE_PRIVATE);// Get the editor to make changesSharedPreferences.Editor editor = sharedPreferences.edit();
// Store the user's nameeditor.putString("name", "John Doe");
// Apply the changeseditor.apply();
You can later retrieve the stored value using the key:
String name = sharedPreferences.getString("name", "");
Shared preferences offer a convenient way to persist small amounts of data across app sessions without the need for a complex database setup.
2. File Storage
Android provides file storage options for storing larger amounts of data or files that need to be accessed directly. File storage can be used to save images, audio files, videos, or any other file type required by your app.
There are two main types of file storage available in Android: internal storage and external storage. Internal storage is private to your app and can only be accessed by your app. External storage, on the other hand, is accessible by other apps and the user, such as the device's SD card.
To save a file in internal storage, you can use the getFilesDir() method to get the directory where your app can write files. You can then create a File object and write data to it. For example:
String filename = "myfile.txt";String fileContents = "Hello, world!";FileOutputStream outputStream;try {outputStream = openFileOutput(filename, Context.MODE_PRIVATE);outputStream.write(fileContents.getBytes());outputStream.close();} catch (Exception e) {e.printStackTrace();}
To save a file in external storage, you need to request the necessary permissions in your app's manifest file. Once you have the necessary permissions, you can use the getExternalFilesDir() method to get the directory where your app can write files. The process of writing data to a file in external storage is similar to internal storage.
File storage provides flexibility in managing and accessing larger amounts of data or files directly, but it requires careful handling and consideration of permissions and security.
3. SQLite Databases
SQLite is a lightweight and efficient relational database engine that is integrated with Android. It provides a way to store and manage structured data in a local database, offering advanced querying capabilities and data manipulation.
SQLite databases in Android are stored as files in the app's private storage area and can be accessed using the SQLiteDatabase class. To work with a SQLite database, you need to define a database schema, which includes tables, columns, and relationships between tables.
The Android SDK provides the SQLiteOpenHelper class, which simplifies the creation and management of SQLite databases. This class helps you handle database creation, versioning, and upgrades, ensuring data consistency and compatibility.
With SQLite, you can perform various operations, such as creating tables, inserting, updating, and deleting records, and executing complex queries using the SQL language. SQLite provides a robust and efficient way to store and retrieve structured data in your Android app.
Handling User Input and Navigation
Effective user input and navigation are essential for creating intuitive and engaging apps. In this section, we will explore various input methods, such as touch gestures and hardware sensors. We will also discuss navigation patterns and techniques to ensure a seamless user experience.
1. User Input Controls
Android offers a wide range of user input controls that allow users to interact with your app. These controls include buttons, text fields, checkboxes, radio buttons, spinners, sliders, and more. Understanding how to handle user input and utilize these controls is crucial for creating interactive and user-friendly apps.
When a user interacts with an input control, you can capture the input and perform appropriate actions based on the user's selections or inputs. For example, you can handle button clicks, retrieve text from text fields, or respond to checkbox selections.
Android provides various event listeners, such as OnClickListener, OnCheckedChangeListener, and OnItemSelectedListener, to handle user input events. By implementing these listeners and attaching them to the respective input controls, you can respond to user actions effectively.
2. Touch Gestures and Gestural Navigation
Touch gestures play a significant role in modern mobile app interaction. Android provides a comprehensive set of touch gesture recognition APIs that allow you to capture and respond to various touch events, such as taps, swipes, pinches, and long presses.
By utilizing touch gestures, you can enhance the user experience and provide more intuitive ways for users to interact with your app. For example, you can implement swipe gestures to navigate between different screens or use pinch gestures for zooming in and out on images or maps.
Gestural navigation has become increasingly popular in Android apps, especially with the introduction of gesture-based navigation systems in newer Android versions. By incorporating gestural navigation patterns, you can provide a seamless and immersive user experience.
It's important to handle touch gestures accurately and provide appropriate feedback to the user. Android provides gesture detectors and listeners, such as GestureDetector and View.OnTouchListener, to simplify the implementation of touch gesture recognition in your app.
3. Hardware Sensors and Contextual Input
Android devices are equipped with various hardware sensors that can provide valuable input for your app. These sensors include accelerometers, gyroscopes, GPS, proximity sensors, ambient light sensors, and more.
By leveraging hardware sensors, you can create innovative and context-aware apps. For example, you can use the accelerometer to detect device movement and implement shake-to-refresh functionality or utilize GPS data to provide location-based services.
Android provides sensor APIs that allow you to access and utilize the data from different hardware sensors. You can register sensor listeners and receive sensor data updates, enabling you to respond and adapt to the user's environment.
4. Navigation Patterns and Techniques
Navigation is a critical aspect of app design, ensuring that users can move between different screens and sections seamlessly. Android offers various navigation patterns and techniques that you can implement to create a cohesive and intuitive navigation flow.
One common navigation pattern is the use of a navigation drawer, which provides access to different app sections or functionalities through a sliding panel. The navigation drawer can be accessed by swiping from the edge of the screen or by tapping on an icon.
Another common navigation pattern is the use of tabs, which allow users to switch between different views or categories within an app. Tabs can be implemented using the TabLayout component, providing a convenient and visually appealing way for users to navigate within your app.
Android also supports bottom navigation, where navigation options are placed at the bottom of the screen. This pattern is especially useful for apps with a small number of primary destinations.
It's important to consider the overall app architecture and user flow when designing the navigation for your app. By providing clear and consistent navigation, you can enhance the user experience and make your app more intuitive to use.
Networking and Web Services
Many modern apps require interaction with web services and APIs. In this section, we will delve into networking fundamentals and demonstrate how to make HTTP requests, handle responses, and parse JSON data. We will also explore popular libraries and frameworks that simplify networking tasks.
1. Networking Fundamentals
Networking is the process of establishing a connection between your app and a remote server to exchange data. In Android, networking is typically done over the HTTP (Hypertext Transfer Protocol) protocol, which is widely used for web communication.
When working with networking in Android, it's important to perform network operations asynchronously to avoid blocking the main UI thread. Android provides the AsyncTask class, which allows you to perform background tasks and update the UI thread when necessary.
To establish a network connection and make HTTP requests, you can use the HttpURLConnection class or third-party libraries like OkHttp or Volley. These libraries provide abstractions and convenience methods for handling network operations, making it easier to interact with web services and APIs.
2. Making HTTP Requests
To make an HTTP request, you need to specify the URL of the server and the desired HTTP method (GET, POST, PUT, DELETE, etc.). You can also include request headers, such as authentication tokens or content type information, and send request parameters or data, if required.
When making an HTTP request, you should handle potential exceptions, such as network connectivity issues or server errors. It's also important to handle different response codes, such as success (200), redirection (3xx), client errors (4xx), or server errors (5xx), and take appropriate actions based on the response.
Here's an example of making a simple GET request using HttpURLConnection:
URL url = new URL("https://api.example.com/data");HttpURLConnection conn = (HttpURLConnection) url.openConnection();conn.setRequestMethod("GET");// Set request headers if necessaryconn.setRequestProperty("Authorization", "Bearer token");
int responseCode = conn.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {// Read the response from the serverBufferedReader reader = new BufferedReader(new InputStreamReader(conn.getInputStream()));String line;StringBuilder response = new StringBuilder();
while ((line = reader.readLine()) != null) {response.append(line);}
reader.close();
// Process the response dataString responseData = response.toString();// ...} else {// Handle error response}
This example demonstrates the basic process of making an HTTP request, handling the response, and reading the response data. Depending on the specific requirements of your app and the API you are interacting with, you may need to include additional headers, send request parameters, or handle different types of responses.
3. Parsing JSON Data
JSON (JavaScript Object Notation) is a widely used data interchange format that is lightweight and easy to read and write. Many web services and APIs return data in JSON format, making it necessary to parse JSON responses in your Android app.
Android provides the JSON library, which allows you to parse JSON data and convert it into Java objects. The library provides classes like JSONObject and JSONArray to represent JSON objects and arrays, respectively.
To parse JSON data, you need to extract the desired values from the JSON structure and convert them into appropriate data types. You can use methods like getString(), getInt(), getBoolean(), or getJSONObject() to retrieve values from JSON objects and arrays.
Here's an example of parsing a JSON response:
String jsonResponse = "{ \"name\": \"John\", \"age\": 30 }";JSONObject jsonObject = new JSONObject(jsonResponse);String name = jsonObject.getString("name");int age = jsonObject.getInt("age");
// Use the retrieved values// ...
In this example, we parse a JSON response containing a name and age value. We extract the values using the getString() and getInt() methods and store them in variables for further use in the app.
4. Popular Networking Libraries
While Android provides native APIs for networking, several third-party libraries can simplify the process and offer additional features. These libraries handle common networking tasks, such as making HTTP requests, handling response parsing, caching, and managing network connections.
Some popular networking libraries for Android include:
- OkHttp: OkHttp is a powerful and efficient HTTP client for Android. It offers features like connection pooling, transparent response compression, request/response interception, and more. OkHttp simplifies the process of making HTTP requests and handles many networking tasks behind the scenes.
- Retrofit: Retrofit is a type-safe HTTP client library that makes it easy to consume RESTful APIs. It provides a high-level interface for defining API endpoints and automatically converts responses to Java objects using JSON parsing libraries like Gson or Moshi.
- Volley: Volley is an easy-to-use networking library provided by Google. It handles asynchronous network requests, response caching, image loading, and more. Volley simplifies the process of making network requests and provides efficient handling of image loading and caching.
These libraries offer a range of features and can significantly reduce the amount of boilerplate code required for networking tasks. Choose the library that best fits your needs and integrates well with your app architecture.
Integrating Multimedia and Device Features
Android devices offer a myriad of features, including cameras, sensors, and multimedia capabilities. In this section, we will discuss how to leverage these features to enhance your app's functionality and user experience. We will cover topics such as capturing photos and videos, playing audio and video files, and utilizing device sensors.
1. Working with the Camera
The camera is one of the most powerful features of Android devices. With the camera, you can capture photos and videos, implement barcode scanning, create augmented reality experiences, and more.
Android provides the Camera API, which allows you to interact with the device's camera hardware and capture images and videos. However, starting from Android 5.0 (Lollipop), the Camera2 API was introduced, offering a more flexible and advanced way to access camera features.
To work with the camera, you need to request the necessary permissions in your app's manifest file and handle runtime permissions on devices running Android 6.0 (Marshmallow) or higher.
Using the Camera2 API, you can implement features like autofocus, flash control, manual exposure settings, and more. You can also capture still images or record videos with various resolutions and quality settings.
Here's an example of capturing a photo using the Camera2 API:
// Initialize camera managerCameraManager cameraManager = (CameraManager) getSystemService(Context.CAMERA_SERVICE);// Get the camera ID (usually the back camera)String cameraId = cameraManager.getCameraIdList()[0];
// Open the cameracameraManager.openCamera(cameraId, new CameraDevice.StateCallback() {@Overridepublic void onOpened(@NonNull CameraDevice camera) {// Camera opened, create a capture session// ...}
@Overridepublic void onDisconnected(@NonNull CameraDevice camera) {// Camera disconnected, release resources// ...}
@Overridepublic void onError(@NonNull CameraDevice camera, int error) {// Camera error, handle accordingly// ...}}, null);
// Create a capture sessionCameraCaptureSession.StateCallback sessionCallback = new CameraCaptureSession.StateCallback() {@Overridepublic void onConfigured(@NonNull CameraCaptureSession session) {try {// Create a capture requestCaptureRequest.Builder captureRequestBuilder = session.getDevice().createCaptureRequest(CameraDevice.TEMPLATE_STILL_CAPTURE);captureRequestBuilder.addTarget(imageReader.getSurface());
// Capture the imagesession.capture(captureRequestBuilder.build(), new CameraCaptureSession.CaptureCallback() {@Overridepublic void onCaptureCompleted(@NonNull CameraCaptureSession session, @NonNull CaptureRequest request, @NonNull TotalCaptureResult result) {// Image captured, process the image// ...}}, null);} catch (CameraAccessException e) {e.printStackTrace();}}
@Overridepublic void onConfigureFailed(@NonNull CameraCaptureSession session) {// Capture session configuration failed, handle accordingly// ...}};
// Create an image readerImageReader imageReader = ImageReader.newInstance(imageWidth, imageHeight, ImageFormat.JPEG, 1);imageReader.setOnImageAvailableListener(new ImageReader.OnImageAvailableListener() {@Overridepublic void onImageAvailable(ImageReader reader) {// Image available, process the image// ...}}, null);
// Create a capture sessioncamera.createCaptureSession(Collections.singletonList(imageReader.getSurface()), sessionCallback, null);
This example demonstrates the basic process of capturing a still image using the Camera2 API. You need to initialize the camera manager, open the camera, create a capture session, and configure the image capture request. Once the image is captured, you can process it further as needed.
2. Playing Audio and Video
Android provides powerful multimedia capabilities, allowing you to play audio and video files in your app. Whether it's playing music, streaming videos, or implementing audio recording, understanding how to work with multimedia is essential for creating engaging and immersive experiences.
To play audio files, you can use the MediaPlayer class provided by Android. MediaPlayer supports various audio formats and provides methods for controlling playback, such as play(), pause(), stop(), and seekTo(). You can load audio files from resources, assets, or the device's storage.
Here's an example of playing an audio file using MediaPlayer:
// Create a MediaPlayer instanceMediaPlayer mediaPlayer = MediaPlayer.create(context, R.raw.audio_file);// Start playbackmediaPlayer.start();
// Pause playbackmediaPlayer.pause();
// Stop playbackmediaPlayer.stop();
// Release resourcesmediaPlayer.release();
For playing video files, Android provides the VideoView class, which simplifies video playback. VideoView handles video rendering and provides methods for controlling playback, similar to MediaPlayer.
Here's an example of playing a video file using VideoView:
// Create a VideoView instanceVideoView videoView = findViewById(R.id.video_view);// Set the video file path or URIvideoView.setVideoPath("/path/to/video.mp4");
// Start playbackvideoView.start();
// Pause playbackvideoView.pause();
// Stop playbackvideoView.stopPlayback();
Android also supports streaming audio and video content over the network using protocols like HTTP Live Streaming (HLS) or Dynamic Adaptive Streaming over HTTP (DASH). You can utilize streaming libraries or build your own streaming solutions depending on your app's requirements.
3. Utilizing Device Sensors
Android devices are equipped with various sensors that can provide valuable input for your app. These sensors include accelerometers, gyroscopes, magnetometers, barometers, GPS, proximity sensors, ambient light sensors, and more.
By leveraging device sensors, you can create innovative and context-aware apps. For example, you can use the accelerometer to detect device movement and implement shake-to-refresh functionality or utilize GPS data to provide location-based services.
Android provides sensor APIs that allow you to access and utilize the data from different hardware sensors. You can register sensor listeners and receive sensor data updates, enabling you to respond and adapt to the user's environment.
Here's an example of registering a sensor listener for the accelerometer:
// Initialize sensor managerSensorManager sensorManager = (SensorManager) getSystemService(Context.SENSOR_SERVICE);// Get the accelerometer sensorSensor accelerometer = sensorManager.getDefaultSensor(Sensor.TYPE_ACCELEROMETER);
// Register sensor listenersensorManager.registerListener(new SensorEventListener() {@Overridepublic void onSensorChanged(SensorEvent event) {float x = event.values[0];float y = event.values[1];float z = event.values[2];// Process accelerometer data// ...}
@Overridepublic void onAccuracyChanged(Sensor sensor, int accuracy) {// Sensor accuracy changed, handle accordingly// ...}}, accelerometer, SensorManager.SENSOR_DELAY_NORMAL);
In this example, we initialize the sensor manager, get the accelerometer sensor, and register a sensor listener. The listener receives updates whenever the accelerometer sensor data changes. You can then process the accelerometer data as needed for your app's functionality.
Other sensors follow a similar registration and data retrieval process. By understanding the capabilities of different sensors and utilizing their data effectively, you can create unique and engaging experiences in your app.
Testing and Debugging Android Apps
Testing and debugging are crucial steps in the app development process. In this section, we will explore various testing techniques and tools available for Android developers. We will cover unit testing, automated UI testing, and debugging techniques to ensure the quality and stability of your app.
1. Unit Testing
Unit testing is the process of testing individual units or components of your app in isolation to verify their correctness. In Android, unit testing is typically done using frameworks like JUnit and Mockito.
Unit tests focus on testing specific methods or classes, checking if they produce the expected output for a given input. By writing unit tests, you can catch bugs and issues early in the development process, ensure code quality, and simplify the debugging process.
Android Studio provides built-in support for unit testing, allowing you to create and run tests directly from the IDE. You can write tests for your app's business logic, data handling, or any other critical functionality.
Here's an example of a unit test using JUnit:
import org.junit.Test;import static org.junit.Assert.assertEquals;public class MyUnitTest {@Testpublic void addition_isCorrect() {int result = 2 + 2;assertEquals(4, result);}}
In this example, we write a simple unit test to verify the correctness of the addition operation. By using the assertEquals() method, we check if the result of 2 + 2 is equal to 4. If the test fails, it indicates an issue with the addition operation.
2. Automated UI Testing
Automated UI testing, also known as instrumented testing, involves testing your app's user interface and interactions automatically. This type of testing ensures that your app functions correctly and provides a smooth user experience.
Android provides the Espresso testing framework, which allows you to write and run UI tests in a simulated environment or on actual devices. Espresso provides a set of APIs that simulate user interactions, such as button clicks, text inputs, and gestures.
With Espresso, you can write tests that cover various user flows and scenarios, ensuring that your app's UI behaves as expected. You can verify if certain UI elements are present, check if specific actions trigger the desired results, and validate the correctness of data displayed in the UI.
Here's an example of an Espresso UI test:
import androidx.test.espresso.Espresso;import androidx.test.espresso.action.ViewActions;import androidx.test.espresso.matcher.ViewMatchers;import androidx.test.ext.junit.runners.AndroidJUnit4;import androidx.test.rule.ActivityTestRule;import org.junit.Rule;import org.junit.Test;import org.junit.runner.RunWith;
@RunWith(AndroidJUnit4.class)public class MyUiTest {@Rulepublic ActivityTestRule<MainActivity> activityRule = new ActivityTestRule<>(MainActivity.class);
@Testpublic void buttonClick_shouldDisplayMessage() {Espresso.onView(ViewMatchers.withId(R.id.button)).perform(ViewActions.click());Espresso.onView(ViewMatchers.withId(R.id.text_view)).check(ViewAssertions.matches(ViewMatchers.withText("Button clicked")));}}
In this example, we write a UI test that checks if clicking a button updates a TextView with the expected text. We use the onView() method to find the button and perform a click action on it. Then, we find the TextView and validate its text using the check() method.
Automated UI testing helps ensure that your app's UI is functional and behaves correctly across different devicesand screen sizes. By covering various user interactions and scenarios, you can catch UI-related issues and ensure a seamless user experience.
3. Debugging Techniques
Debugging is the process of identifying and fixing issues in your app's code. Android provides several debugging tools and techniques to help you track down and resolve bugs efficiently.
One of the primary tools for debugging in Android is the Android Debug Bridge (ADB). ADB allows you to connect to a device or emulator and execute various debugging commands. With ADB, you can view logs, capture screenshots, inspect app components, and more.
Android Studio also provides a powerful integrated debugger. You can set breakpoints in your code, step through the execution, inspect variables and objects, and analyze the app's state during runtime. The debugger helps you identify issues, understand program flow, and validate your assumptions.
Additionally, Android Studio offers the Logcat tool, which displays logs generated by your app and the Android system. Logs are valuable for debugging and understanding the behavior of your app. You can log messages at different levels, such as verbose, debug, info, warning, or error, and filter logs based on tags or priorities.
To use the Logcat tool, you can use the Log class provided by Android. For example:
import android.util.Log;public class MyClass {private static final String TAG = "MyClass";
public void doSomething() {Log.d(TAG, "Doing something...");// ...}}
In this example, we log a debug message using the Log.d() method. The first parameter is the log tag, which helps identify the source of the log message. The second parameter is the log message itself.
When debugging your app, it's also important to gather user feedback and collect crash reports. User feedback can provide valuable insights into app issues and usability problems. Crash reports help identify crashes and exceptions occurring in your app, allowing you to prioritize and fix critical issues.
Several crash reporting tools, such as Firebase Crashlytics or Bugsnag, can automatically collect crash reports and provide detailed crash logs and stack traces. These tools help streamline the debugging process and ensure that critical issues are addressed promptly.
Publishing and Monetizing Your Android App
Once you have developed and tested your app, it's time to publish it on the Google Play Store. In this section, we will guide you through the app submission process, including creating a developer account, preparing your app listing, and optimizing for discoverability. We will also discuss different monetization strategies to generate revenue from your app.
1. Creating a Developer Account
Before you can publish your app on the Google Play Store, you need to create a developer account. A developer account allows you to manage and publish your apps, access user reviews and ratings, and track app performance and analytics.
To create a developer account, follow these steps:
- Go to the Google Play Console website (https://play.google.com/apps/publish/).
- Sign in with your Google account or create a new one.
- Agree to the terms and conditions and pay the one-time developer registration fee (if applicable).
- Complete your developer profile, including your developer name, email address, and contact details.
- Verify your account by providing a phone number for SMS verification.
Once your developer account is created, you can start preparing your app for submission.
2. Preparing Your App Listing
Before submitting your app, you need to prepare your app listing, which includes all the necessary information and assets for your app's store page. A well-crafted app listing helps attract users and provides essential details about your app's features and functionality.
Here are some key elements to consider when preparing your app listing:
- App Title: Choose a catchy and descriptive title that accurately represents your app.
- App Description: Write a compelling and concise description of your app's features, benefits, and value proposition. Highlight unique selling points and key functionalities.
- Screenshots and Videos: Include high-quality screenshots and videos that showcase your app's interface, features, and user experience. Use compelling visuals to grab users' attention.
- App Icon: Design an appealing and recognizable app icon that reflects your brand or app's purpose. The app icon should be in a square shape and meet the specified size requirements.
- App Categorization: Choose the most appropriate category and subcategory for your app to ensure it appears in relevant search results and app recommendations.
- Keywords: Select relevant keywords that describe your app and its features. Use these keywords strategically in your app's title, description, and other metadata to improve discoverability.
- Localization: Consider localizing your app listing to reach a broader audience. Translate your app's title, description, and screenshots into different languages to cater to users from various regions.
Ensure that your app listing adheres to the Google Play Store's guidelines and policies. Avoid using misleading information, inappropriate content, or copyrighted materials in your app listing.
3. Optimizing for Discoverability
With millions of apps available on the Google Play Store, optimizing your app's discoverability is crucial for attracting users. To increase your app's visibility and chances of being found, consider implementing the following strategies:
- App Store Optimization (ASO): ASO involves optimizing your app's metadata, including title, description, keywords, and visuals, for higher search rankings. Research relevant keywords and incorporate them naturally into your app's listing to improve its visibility in search results.
- User Ratings and Reviews: Encourage users to rate and review your app. Positive ratings and reviews not only boost your app's reputation but also influence user decision-making. Respond to user feedback, address concerns, and continuously improve your app based on user suggestions.
- Promotion and Marketing: Develop a marketing strategy to promote your app and reach your target audience. Utilize social media platforms, blogs, and other marketing channels to create awareness and generate interest in your app. Collaborate with influencers or app review websites to gain exposure.
- App Updates and Maintenance: Regularly update your app with new features, bug fixes, and performance improvements. Keeping your app up to date not only enhances user experience but also signals to the Google Play Store that your app is actively maintained and supported.
By implementing these strategies, you can increase your app's visibility and attract more users to download and use your app.
4. Monetization Strategies
Monetizing your app allows you to generate revenue from your hard work and investment. There are several monetization strategies you can consider, depending on your app's nature and target audience:
- In-App Purchases: Offer additional features, content, or virtual goods for purchase within your app. Implement in-app purchase functionality using the Google Play Billing Library to handle transactions securely.
- Advertisements: Integrate ads into your app using ad networks like AdMob. Display banner ads, interstitial ads, or rewarded video ads to earn revenue based on impressions or user interactions.
- Subscriptions: Implement subscription-based models, offering users access to premium content or services on a recurring basis. Utilize the Google Play Billing Library to manage subscription payments and renewals.
- Freemium Model: Offer a free version of your app with limited features or content and provide an option to upgrade to a premium version for a one-time fee or subscription.
- Sponsorships and Partnerships: Collaborate with brands or businesses that align with your app's target audience. Offer sponsorship opportunities or partnerships to promote their products or services within your app.
Choose a monetization strategy that aligns with your app's value proposition and user experience. Consider the preferences and behaviors of your target audience when deciding on the most suitable approach.
It's essential to balance monetization with user experience and avoid intrusive or excessive ads that may negatively impact the user's perception of your app. Strive to provide value to your users and build a loyal user base.
Continuous Learning and Staying Updated
Android app development is a dynamic field, constantly evolving with new technologies and updates. In this final section, we will provide you with resources and strategies to stay updated on the latest trends, best practices, and platform changes. We will explore online communities, forums, and official documentation to help you continue your learning journey beyond this guide.
1. Online Communities and Forums
Engaging with the Android developer community is a great way to stay updated, learn from others, and seek guidance. Join online communities and forums dedicated to Android development, such as Reddit's r/androiddev, Stack Overflow, or the official Android Developers community.
Participate in discussions, ask questions, and share your knowledge and experiences. By being an active member of these communities, you can learn from others, gain insights into industry trends, and stay updated on the latest developments in Android app development.
2. Official Documentation and Blogs
The official Android Developers website (https://developer.android.com/) is a valuable resource for learning about Android app development. Explore the documentation, guides, and tutorials provided by Google to gain in-depth knowledge about various topics, APIs, and best practices.
In addition to the official documentation, Google's Android Developers Blog (https://android-developers.googleblog.com/) provides regular updates, announcements, and insights from the Android team. Stay subscribed to the blog to receive notifications about new releases, updates, and important information related to Android app development.
3. Online Courses and Tutorials
Online learning platforms offer a wide range of courses and tutorials specifically tailored to Android app development. Platforms like Udacity, Coursera, and Pluralsight offer comprehensive courses taught by industry experts.
Take advantage of these courses to deepen your understanding of Android app development, learn new techniques, and expand your skillset. Follow along with practical projects and exercises to apply your knowledge in real-world scenarios.
4. Attend Conferences and Meetups
Conferences and meetups provide opportunities to connect with fellow developers, industry experts, and thought leaders in the Android community. Attend events like Google I/O, Droidcon, or local developer meetups to network, learn from inspiring talks and workshops, and stay up to date with the latest trends and technologies.
5. Experiment and Build Personal Projects
One of the best ways to learn and improve your Android development skills is by building personal projects. Experiment with different ideas, try out new libraries and frameworks, and challenge yourself to solve unique problems.
Building personal projects allows you to apply your knowledge, gain hands-on experience, and overcome practical challenges. It also provides a platform to showcase your skills and create a portfolio that can impress potential employers or clients.
Remember to continuously practice and refine your skills through regular coding sessions. Stay curious, explore new ideas, and never stop learning.
In conclusion, the Android App Developer Program offers a wealth of resources and support for developers looking to create innovative and successful Android apps. By mastering the fundamentals, exploring advanced techniques, and staying informed about the latest updates, you can unlock endless possibilities in the world of Android app development. So, what are you waiting for? Start your Android app development journey today!